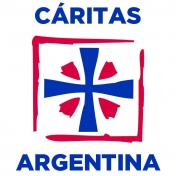
Detalle del Proyecto
EDUCACIÓN y PRIMERA INFANCIA
La educación es una herramienta fundamental para la integración social y la equidad. En Cáritas, convocamos al encuentro y al aprendizaje a través del apoyo escolar, talleres familiares y deportivos, voluntariado juvenil, otorgamiento de becas de estudio y distintas actividades que favorecen la creación de amplias redes de contención para el cuidado de la vida desde una perspectiva integral.
Quienes acceden a la educación, se convierten en protagonistas de la transformación de su propia realidad. Colaborá para que podamos seguir trabajando por la inclusión educativa.
**HelpArgentina el el capítulo del Portal para la Inversión Social en América Latina (PILAS) focalizado en Argentina. PILAS está registrado en Nueva York como una organización no gubernamental bajo el artículo 501(c.3) del código impositivo de Estados Unidos (EIN 550790450). Como tal, las donaciones son deducibles de impuestos en toda la extensión de la ley.